The SIP
class constructs objects that represent a single "SiP" or "System-In-Package" module (eg. IMU, MEMS) attached to the physical board. An SiP may be made up of several components like Accelerometers, Gyroscopes, Thermometers, and Compass/Magnetometers.
Supported SiP sensor modules:
- BMP180
- BMP280
- BME280
- HTU21D
- HIH6130
- MPL115A2
- MPL3115A2
- SI7020
- SI7021
- MS5611
- TH02
- DHT11 (Via I2C Backpack)
- DHT21 (Via I2C Backpack)
- DHT22 (Via I2C Backpack)
This list will continue to be updated as more component support is implemented.
Parameters
General Options
Property | Type | Value/Description | Default | Required |
controller | string | BMP180, BMP280, BME280, HTU21D, HIH6130, MPL115A2, MPL3115A2, SI7020, SI7021, MS5611, TH02. The Name of the controller to use | | yes |
freq | Number | In milliseconds, how often data events should fire | 20 | no |
In addition, anything in the options
object passed to the SIP
constructor will be passed along to each of its constituent component sensors (e.g. Thermometer
, Altimeter
, etc.). See relevant sensor component class documentation for more details about what options each takes.
Shape
Some of these properties may or may not exist depending on whether the multi sensor supports it.
Property Name | Description | Read Only |
accelerometer | An instance of Accelerometer class. | Yes |
altimeter | An instance of Altimeter class. | Yes |
barometer | An instance of Barometer class. | Yes |
gyro | An instance of Gyro class. | Yes |
hygrometer | An instance of Hygrometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
temperature | An instance of Thermometer class. | Yes |
Component Initialization
BMP180
new five.SIP({
controller: "BMP180"
});
Property Name | Description | Read Only |
barometer | An instance of Barometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
temperature | An instance of Thermometer class. | Yes |
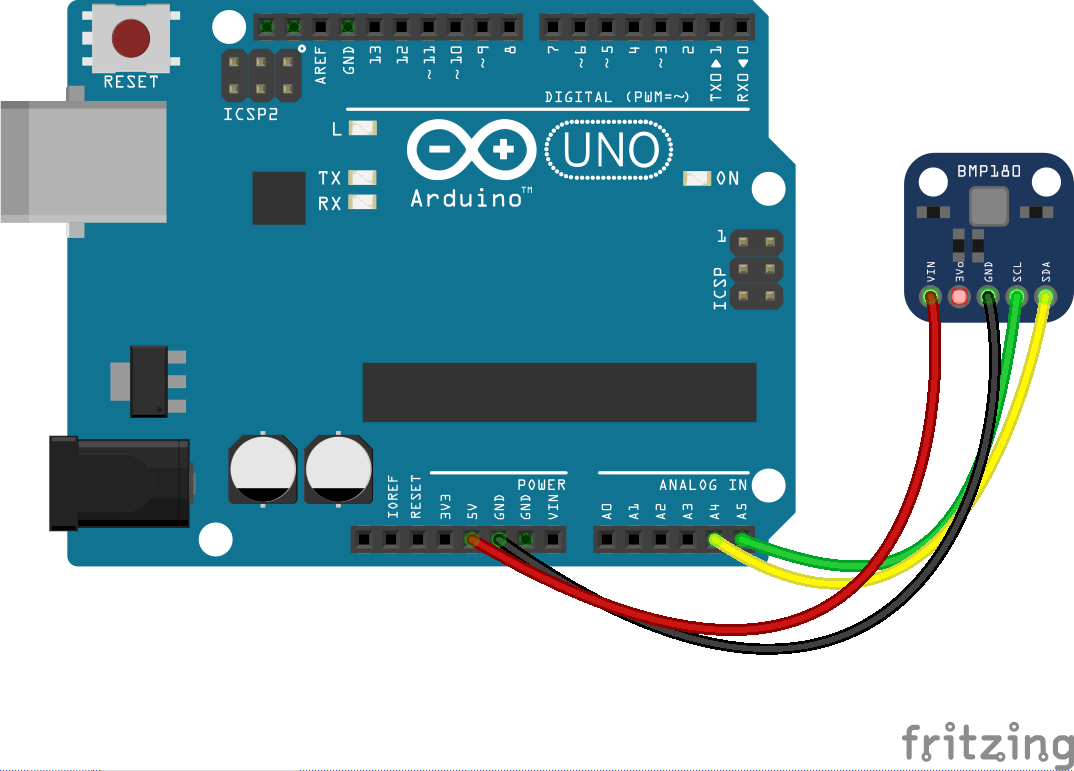
HTU21D
new five.SIP({
controller: "HTU21D"
});
Shape
Property Name | Description | Read Only |
hygrometer | An instance of Hygrometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
temperature | An instance of Thermometer class. | Yes |
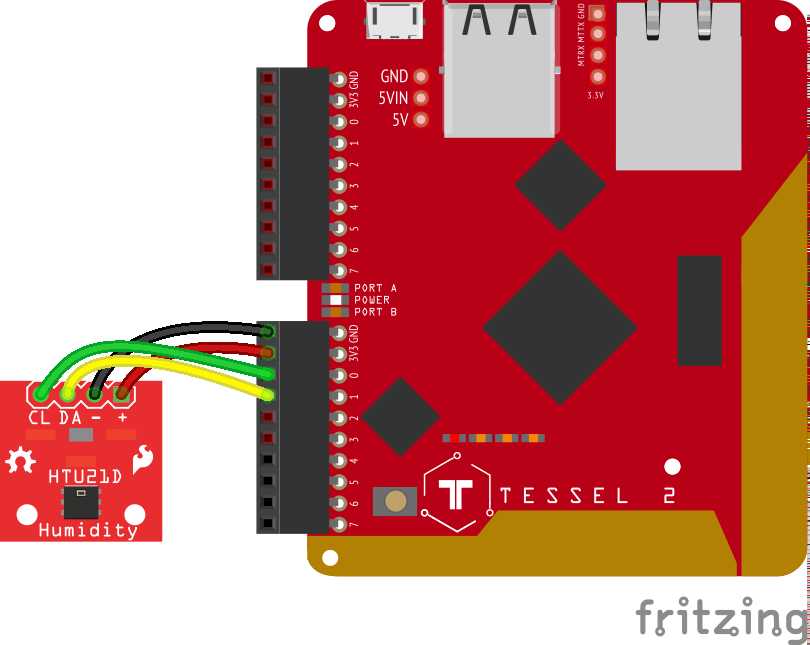
HIH6130
new five.SIP({
controller: "HIH6130"
});
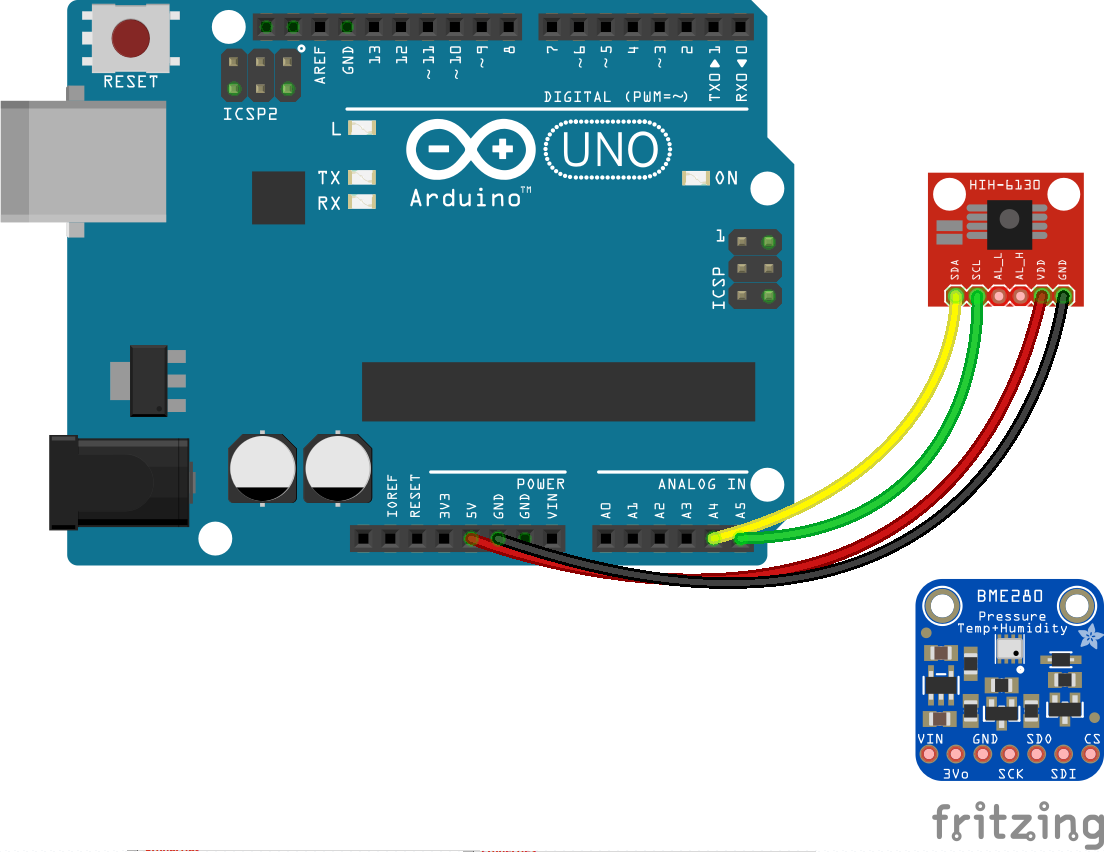
MPL115A2
new five.SIP({
controller: "MPL115A2"
});
Property Name | Description | Read Only |
barometer | An instance of Barometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
temperature | An instance of Thermometer class. | Yes |
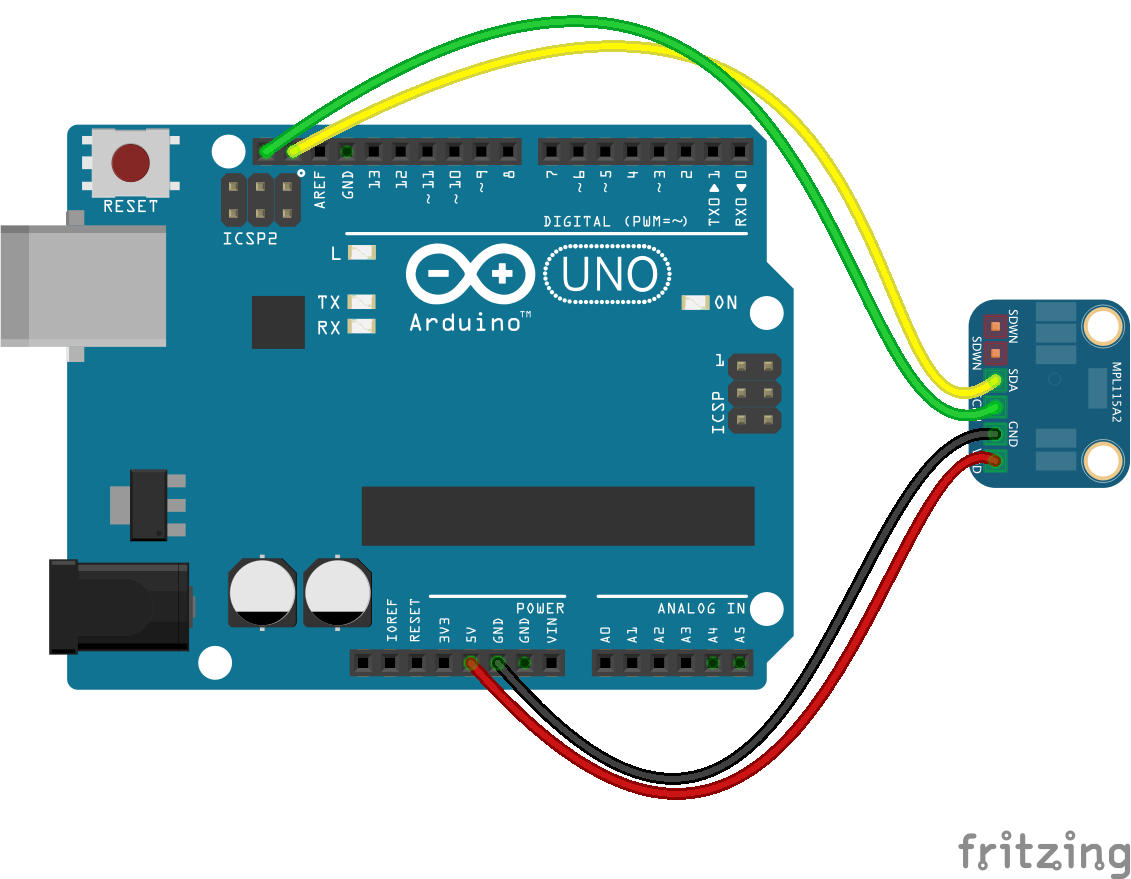
MPL3115A2
new five.SIP({
controller: "MPL3115A2"
});
Property Name | Description | Read Only |
altimeter | An instance of Altimeter class. | Yes |
barometer | An instance of Barometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
temperature | An instance of Thermometer class. | Yes |
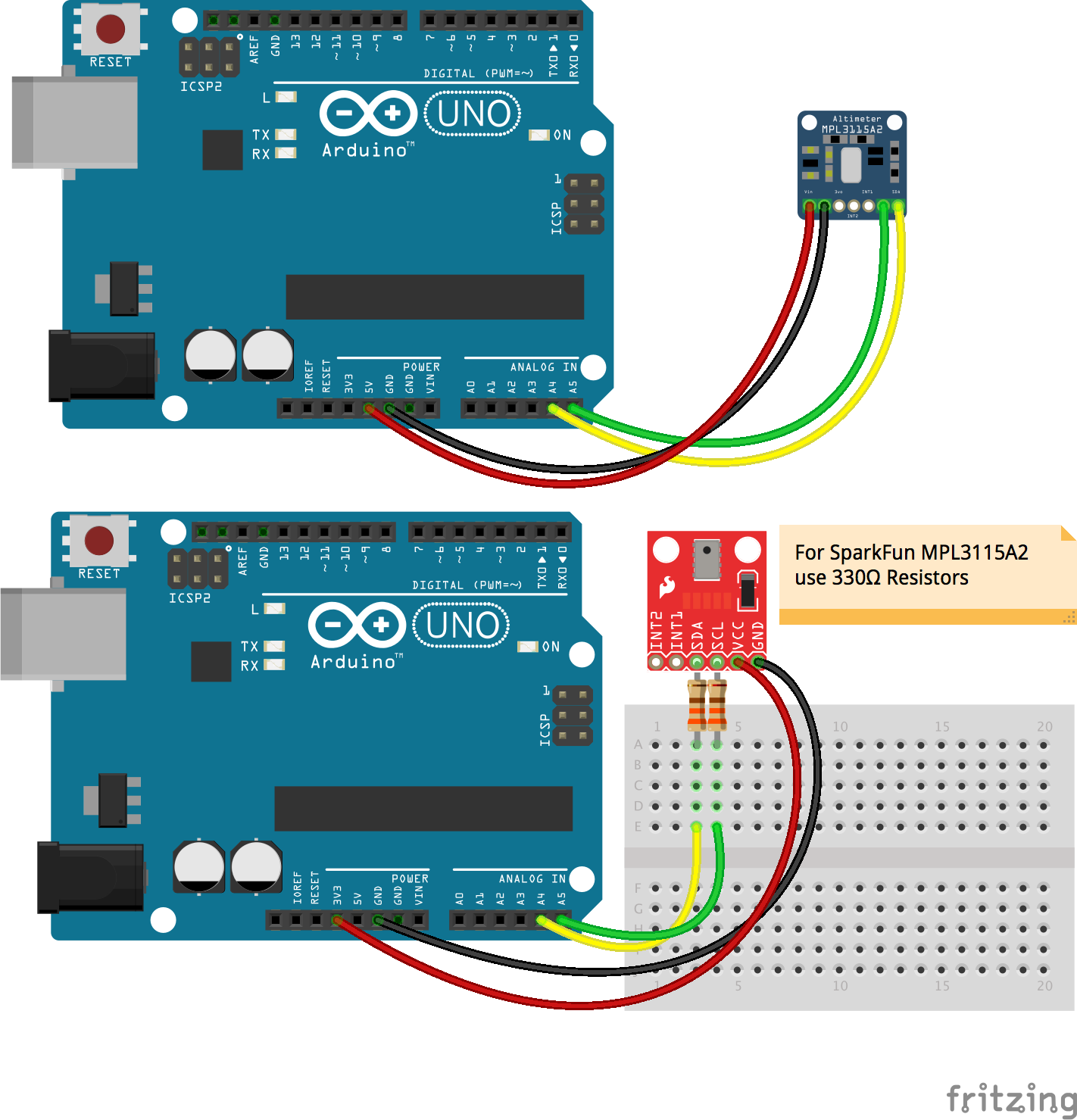
SI7020
new five.SIP({
controller: "SI7020"
});
Property Name | Description | Read Only |
hygrometer | An instance of Hygrometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
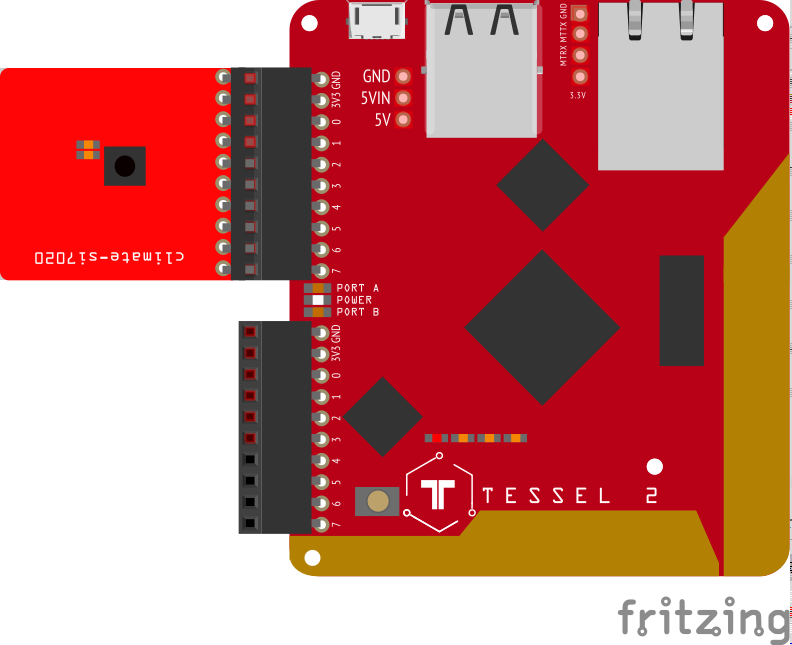
SI7021
new five.SIP({
controller: "SI7021"
});
Property Name | Description | Read Only |
hygrometer | An instance of Hygrometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
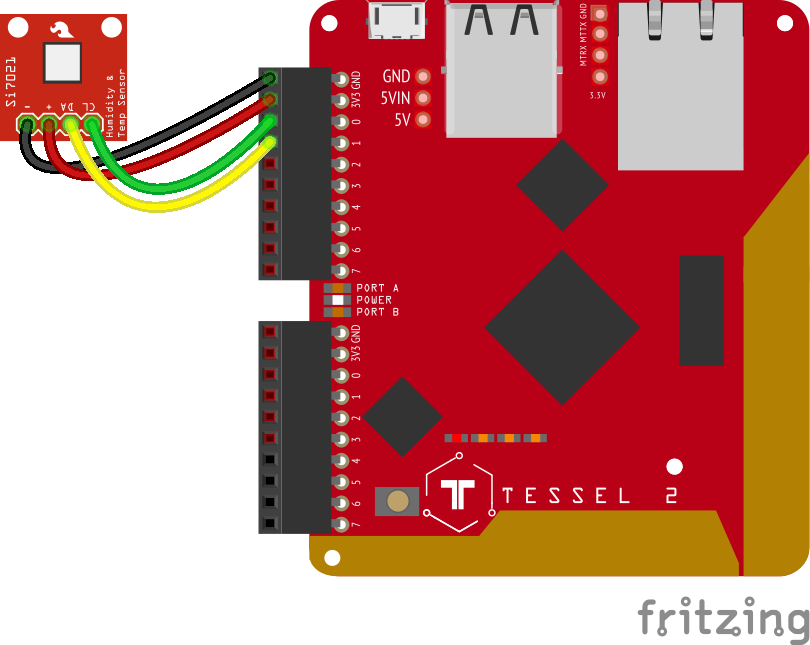
MS5611
new five.SIP({
controller: "MS5611"
});
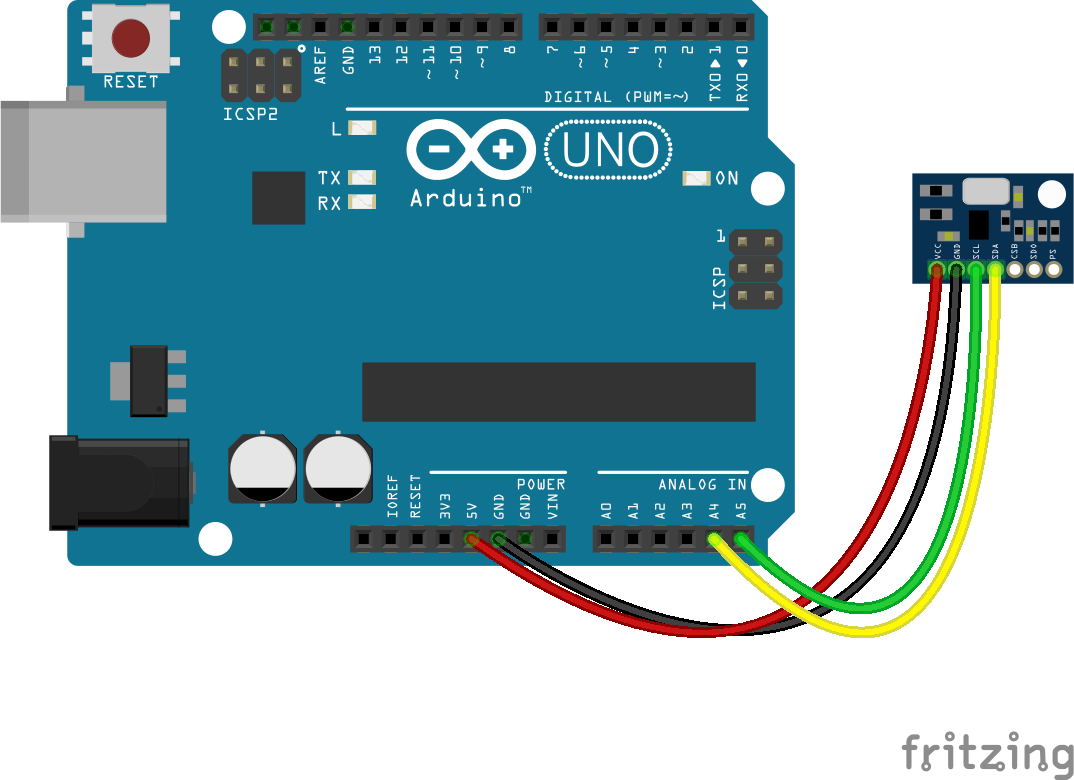
Property Name | Description | Read Only |
altimeter | An instance of Altimeter class. | Yes |
barometer | An instance of Barometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
TH02
new five.SIP({
controller: "TH02"
});
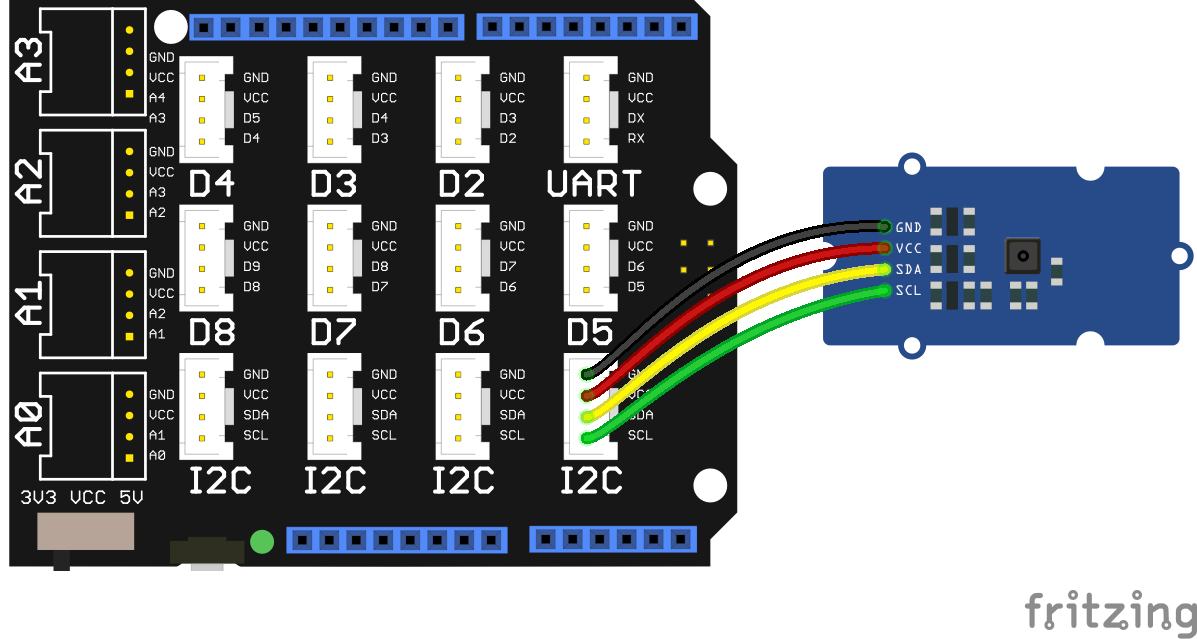
Property Name | Description | Read Only |
hygrometer | An instance of Hygrometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
DHT11_I2C_NANO_BACKPACK
new five.SIP({
controller: "DHT11_I2C_NANO_BACKPACK"
});
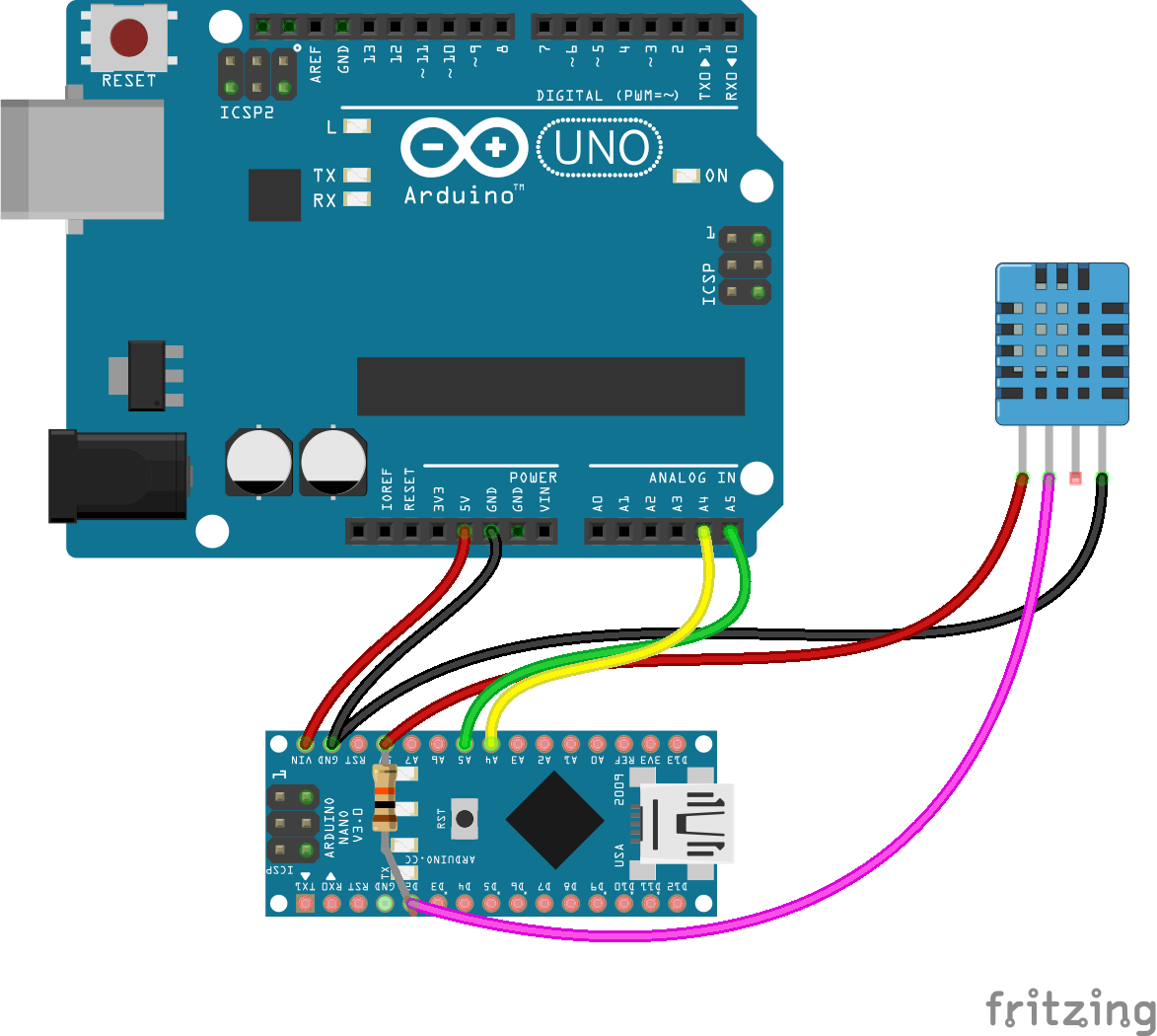
Property Name | Description | Read Only |
hygrometer | An instance of Hygrometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
DHT21_I2C_NANO_BACKPACK
new five.SIP({
controller: "DHT21_I2C_NANO_BACKPACK"
});
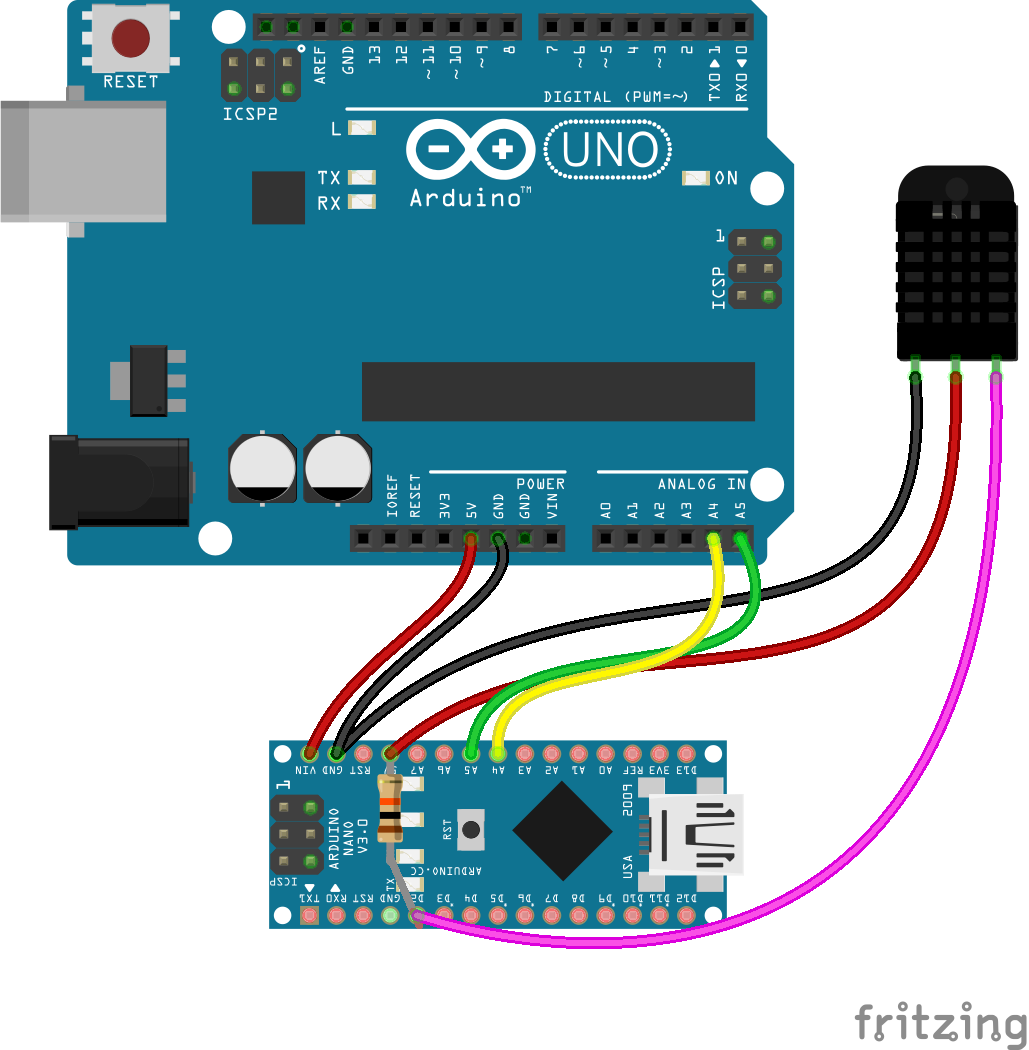
Property Name | Description | Read Only |
hygrometer | An instance of Hygrometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
DHT22_I2C_NANO_BACKPACK
new five.SIP({
controller: "DHT22_I2C_NANO_BACKPACK"
});
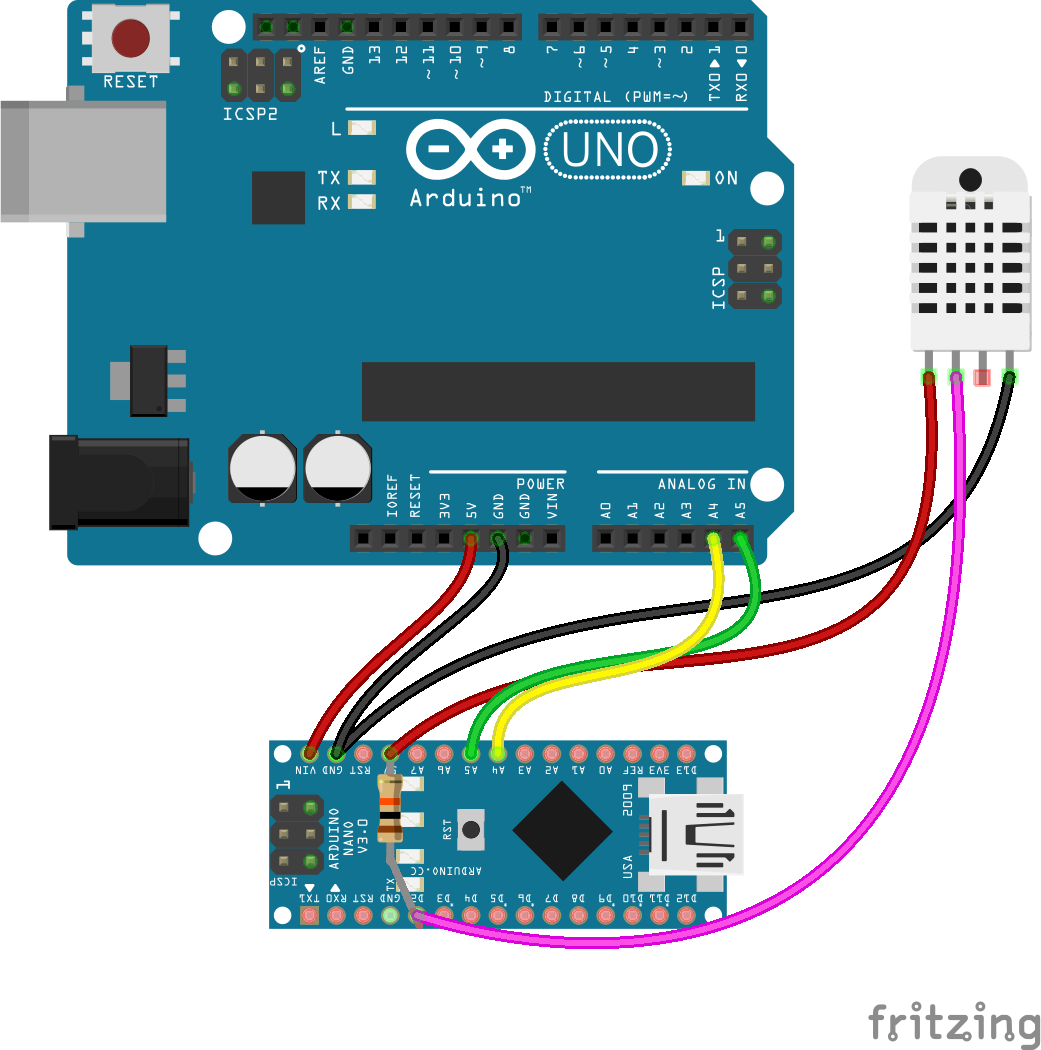
Property Name | Description | Read Only |
hygrometer | An instance of Hygrometer class. | Yes |
thermometer | An instance of Thermometer class. | Yes |
Usage
const five = require("johnny-five");
const board = new five.Board();
board.on("ready", () => {
const sip = new five.SIP({
controller: "MPL115A2"
});
sip.on("data", () => {
console.log("Barometer: %d", sip.barometer.pressure);
console.log("Thermometer: %d", sip.thermometer.celsius);
});
});
const five = require("johnny-five");
const board = new five.Board();
board.on("ready", () => {
const sip = new five.SIP({
controller: "HTU21D"
});
sip.on("change", () => {
console.log("Thermometer");
console.log(" celsius : ", sip.thermometer.celsius);
console.log(" fahrenheit : ", sip.thermometer.fahrenheit);
console.log(" kelvin : ", sip.thermometer.kelvin);
console.log("--------------------------------------");
console.log("Hygrometer");
console.log(" relative humidity : ", sip.hygrometer.relativeHumidity);
console.log("--------------------------------------");
});
});
API
The SIP class does not have an explicit API. Refer to the individual components for their APIs
Events
Note: You may also bind to events on SIP
object component sensors directly, e.g. sip.thermometer.on('change')
0